In Linux, mastering the cpp command is essential for any programmer or developer. Whether you’re a seasoned expert or just starting out, understanding how cpp works can greatly enhance your coding capabilities. With its powerful features and versatility, cpp allows you to preprocess C and C++ source code, making it an indispensable tool for optimizing and debugging your programs.
How to compile C++ code using the cpp command
When it comes to compiling C++ code in Linux, the cpp command plays a crucial role in the preprocessing stage. To compile your C++ code using cpp, you need to follow a few simple steps.
First, open your terminal and navigate to the directory where your C++ source code is located. Once you’re in the correct directory, you can use the cpp command followed by the name of your source file to preprocess it. For example, if your source file is named main.cpp, you would run the following command:
cpp main.cpp
This command will preprocess your code and display the output on the terminal. However, if you want to save the preprocessed code to a separate file, you can redirect the output to a file using the > operator. For instance, to save the preprocessed code to a file named preprocessed.cpp, you would use the following command:
cpp main.cpp > preprocessed.cpp
By following these simple steps, you can compile your C++ code using the cpp command and preprocess it for further optimization and debugging.
Understanding the preprocessing phase in C++
The preprocessing phase in C++ is a crucial step that occurs before the actual compilation of the code. During this phase, the cpp command processes the C++ source code and performs various operations such as macro expansion, inclusion of header files, and conditional compilation.
One of the key functionalities of the cpp command is macro expansion. Macros in C++ allow you to define reusable code snippets that can be expanded during the preprocessing phase. This can greatly improve code readability and maintainability. The cpp command identifies macros in your code and replaces them with their corresponding definitions.
Another important aspect of the preprocessing phase is the inclusion of header files. Header files contain declarations and definitions that are used by multiple source files. The cpp command handles the inclusion of header files by copying their content into the source code at the specified locations. This ensures that the necessary declarations and definitions are available to the compiler during the compilation phase.
Furthermore, the cpp command enables conditional compilation, which allows you to include or exclude certain portions of code based on specified conditions. This can be particularly useful when dealing with platform-specific code or when you want to enable/disable certain features depending on compile-time parameters.
By understanding the preprocessing phase in C++ and utilizing the functionalities of the cpp command, you can effectively optimize and customize your code to meet specific requirements.
Using directives and macros with cpp command
Directives and macros are powerful tools that can be used in conjunction with the cpp command to enhance your coding experience. By utilizing directives and macros, you can effectively control the behavior of the cpp command and customize the preprocessing process according to your needs.
Directives in C++ are instructions that guide the cpp command on how to preprocess the code. They are typically written as preprocessor directives and start with a # symbol. Some commonly used directives include #include, #define, and #ifdef.
The #include directive is used to include header files in your code. By specifying the name of the header file after the #include directive, you can import the necessary declarations and definitions into your source code. This allows for modular programming and improves code reusability.
The #define directive is used to define macros in your code. Macros are essentially placeholders that can be replaced with their corresponding definitions during the preprocessing phase. By using the #define directive, you can create macros and specify their replacement text.
Conditional directives such as #ifdef, #ifndef, and #endif are used to selectively include or exclude certain portions of code based on specified conditions. This can be particularly useful when dealing with platform-specific code or when you want to enable/disable certain features depending on compile-time parameters.
In addition to directives, macros can be defined and utilized with the cpp command to improve code readability and maintainability. Macros allow you to define reusable code snippets that can be expanded during the preprocessing phase, reducing the need for repetitive code.
By leveraging directives and macros in conjunction with the cpp command, you can customize the preprocessing process and optimize your code for better performance and maintainability.
Debugging and error handling with cpp
Debugging and error handling are critical aspects of software development, and the cpp command provides several features to assist you in these areas. By utilizing the debugging and error handling functionalities of cpp, you can effectively identify and resolve issues in your code, saving time and effort.
One of the key features of the cpp command is the ability to generate debug information during the preprocessing phase. Debug information includes line numbers, file names, and other relevant details that can help you pinpoint the exact location of an error or issue in your code. By enabling debug information generation, you can improve the accuracy and efficiency of your debugging process.
Another useful feature provided by the cpp command is the ability to display warnings and errors. When preprocessing your code, cpp performs various checks and validations to ensure that your code is syntactically correct and adheres to the specified standards. If any issues or potential problems are detected, cpp displays appropriate warnings or errors, allowing you to take corrective action.
Furthermore, the cpp command provides options to control the level of verbosity during the preprocessing process. By specifying the desired verbosity level, you can customize the amount of output generated by cpp and focus on the specific details that are relevant to your debugging or error handling tasks.
By utilizing the debugging and error handling features of the cpp command, you can effectively identify and resolve issues in your code, ensuring that your programs are robust and error-free.
Advanced features of the cpp command
While the basic functionalities of the cpp command are essential for preprocessing C++ code, there are also advanced features that can further enhance your coding experience. By exploring these advanced features, you can unlock the full potential of the cpp command and optimize your programming process.
One advanced feature of the cpp command is the ability to define and use predefined macros. Predefined macros are macros that are automatically defined by the cpp command and provide information about the environment and compiler being used. These macros can be utilized in your code to enable platform-specific optimizations or to conditionally compile certain portions of code.
Another advanced feature of the cpp command is the ability to control the behavior of the preprocessor using command-line options. By specifying different options when invoking the cpp command, you can customize the preprocessing process and achieve specific objectives. Some commonly used options include -D to define macros, -U to undefine macros, and -I to specify additional directories to search for header files.
Additionally, the cpp command supports the use of so-called “pragma” directives. Pragmas are compiler-specific instructions that can be used to control various aspects of the compilation process. By utilizing pragma directives with the cpp command, you can fine-tune the behavior of the preprocessor and optimize your code for specific compiler optimizations or platform requirements.
By exploring and utilizing these advanced features of the cpp command, you can take your coding capabilities to the next level and achieve greater efficiency and effectiveness in your programming endeavors.
Common use cases for the cpp command in Linux
The cpp command in Linux offers a wide range of functionalities and can be utilized in various scenarios to optimize and streamline your coding process. Here are some common use cases where the cpp command can prove to be invaluable:
- Conditional Compilation: The cpp command allows you to selectively include or exclude certain portions of code based on specified conditions. This can be particularly useful when dealing with platform-specific code or when you want to enable/disable certain features depending on compile-time parameters.
- Macro Expansion: Macros in C++ allow you to define reusable code snippets that can be expanded during the preprocessing phase. By utilizing macros with the cpp command, you can improve code readability and maintainability, reducing the need for repetitive code.
- Debugging and Error Handling: The cpp command provides features to generate debug information, display warnings and errors, and control the verbosity level of the preprocessing process. By leveraging these features, you can effectively identify and resolve issues in your code, ensuring that your programs are robust and error-free.
- Customization and Optimization: The cpp command offers advanced features such as predefined macros, command-line options, and pragma directives. By exploring and utilizing these advanced features, you can customize the preprocessing process and optimize your code for specific requirements, achieving greater efficiency and effectiveness in your programming endeavors.
By understanding and utilizing the various functionalities of the cpp command, you can tackle a wide range of programming challenges and optimize your coding process for better results.
Best practices for using cpp in C++ development
When it comes to using the cpp command in C++ development, following best practices can greatly enhance your coding experience and ensure that your programs are efficient, maintainable, and error-free. Here are some best practices to keep in mind:
- Use Meaningful Macros: When defining macros, choose names that accurately represent their purpose and functionality. This will improve code readability and make it easier for other developers to understand and maintain your code.
- Avoid Macro Abuse: While macros can be powerful tools, they should be used judiciously. Avoid excessive use of macros and instead favor the use of functions or templates where appropriate. This will make your code more modular and easier to understand.
- Use Conditional Compilation Sparingly: Conditional compilation can be a powerful technique, but it can also make your code more complex and harder to maintain. Use conditional compilation sparingly and only when necessary. Consider alternative approaches such as runtime configuration or template specialization where applicable.
- Enable Debug Information Generation: Enabling debug information generation during the preprocessing phase can greatly aid in the debugging process. By including line numbers, file names, and other relevant details in the debug information, you can quickly pinpoint the location of errors or issues in your code.
- Pay Attention to Warnings and Errors: When preprocessing your code with the cpp command, pay close attention to any warnings or errors that are displayed. Addressing these warnings and errors promptly can help prevent potential issues and ensure that your code is syntactically correct and adheres to the specified standards.
- Experiment with Advanced Features: Don’t be afraid to experiment with the advanced features of the cpp command. Predefined macros, command-line options, and pragma directives can offer powerful customization and optimization capabilities. By exploring these advanced features, you can unlock the full potential of the cpp command and achieve greater efficiency in your programming endeavors.
By following these best practices, you can make the most out of the cpp command in your C++ development projects and ensure that your code is robust, maintainable, and optimized for performance.
Alternatives to the cpp command in Linux
While the cpp command is a powerful tool for preprocessing C++ code in Linux, there are also alternative approaches that you can consider based on your specific requirements and preferences. Here are some popular alternatives to the cpp command:
- Integrated Development Environments (IDEs): Many modern IDEs provide built-in support for preprocessing C++ code. IDEs such as Visual Studio Code, CLion, and Eclipse offer advanced code editing and preprocessing capabilities, making them suitable alternatives to the cpp command.
- Build Systems: Build systems like CMake and Make can also handle the preprocessing of C++ code. These build systems provide extensive control over the compilation and preprocessing process, allowing for greater customization and optimization.
- Preprocessor Libraries: If you require more fine-grained control over the preprocessing process, you can consider using preprocessor libraries such as Boost.Preprocessor or POCO Preprocessor. These libraries offer additional functionalities and flexibility compared to the cpp command.
- Compiler-specific Preprocessors: Some compilers provide their own preprocessor implementations that offer additional features and optimizations. For example, the GNU Compiler Collection (GCC) provides the gcc -E command, which preprocesses C++ code using the GCC compiler’s built-in preprocessor.
When choosing an alternative to the cpp command, consider factors such as the complexity of your code, the level of customization required, and the specific features and optimizations offered by the alternative approach.
Conclusion
In this article, we have explored the powerful cpp command in Linux and its various functionalities. From compiling C++ code to understanding the preprocessing phase, utilizing directives and macros, and leveraging advanced features, the cpp command offers a wide range of capabilities that can greatly enhance your coding experience.
By mastering the cpp command and following best practices, you can optimize your code, improve maintainability, and streamline your programming process. Whether you’re looking to optimize your code or gain a deeper understanding of the preprocessing stage, the cpp command in Linux is an invaluable tool that should not be overlooked.
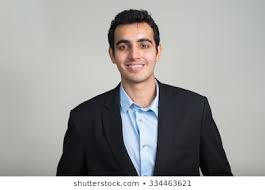
Nishant Verma is a senior web developer who love to share his knowledge about Linux, SysAdmin, and more other web handlers. Currently, he loves to write as content contributor for ServoNode.