Are you tired of getting lost in the file paths while working with Python? Well, worry no more! In this article, we will guide you on how to effortlessly get and change the working directory in Python, saving you time and frustration.
Python is a versatile programming language, widely used for various purposes, including web development and data analysis. Understanding how to navigate through different directories is essential for efficient coding. By mastering the technique of getting and changing the working directory, you will be able to access and manipulate files easily.
We will walk you through the process step by step, explaining different methods and providing examples along the way. Whether you are a beginner or an experienced Python developer, this article will equip you with the skills needed to effectively manage your working directory.
So, if you’re ready to enhance your Python coding workflow and streamline your file operations, let’s dive into the world of working directories in Python!
Why it is important to understand and change working directories?
Before we delve into the details of getting and changing the working directory in Python, let’s first understand why it is essential to grasp this concept.
When working on a Python project, you often need to access files that are stored in different directories. It can become cumbersome and time-consuming to provide the full path to these files every time you want to access them. By understanding how to change the working directory, you can set a default directory where Python will look for files, making your code more concise and readable.
Furthermore, changing the working directory allows you to organize your project’s files and directories in a way that makes sense to you. You can create a logical structure and easily navigate through different directories without getting lost. This not only improves your productivity but also ensures that your code is more maintainable in the long run.
In summary, understanding and changing the working directory in Python is crucial for efficient file operations, code readability, and project organization. By mastering this skill, you will be able to save time, reduce errors, and enhance your overall coding experience.
How to get the current working directory in Python?
Now that we understand the importance of managing the working directory, let’s start by learning how to get the current working directory in Python.
Python provides a built-in function called os.getcwd() that returns the current working directory as a string. This function relies on the operating system to determine the current directory. Let’s see an example:
```python import os current_directory = os.getcwd() print(current_directory) ```
The above code will output the current working directory. You can use this information to verify if you are in the right directory before performing any file operations.
It’s worth noting that the working directory can change during the execution of a Python script. For instance, if you run the script from a different directory, the working directory will be updated to the directory where the script is located. Therefore, it’s essential to keep track of the current working directory if your script relies on specific file paths.
Changing the working directory in Python
Now that we know how to get the current working directory, let’s move on to changing the working directory in Python.
Python provides another built-in function called os.chdir(path) that allows you to change the working directory to the specified path. The path argument can be either an absolute path or a relative path.
To change the working directory to an absolute path, you need to provide the complete path starting from the root directory. Here’s an example:
```python import os new_directory = "/path/to/new/directory" os.chdir(new_directory) ```
In the above code, we use the os.chdir() function to change the working directory to the specified absolute path. After executing this code, any file operations will be performed relative to the new directory.
Alternatively, you can also change the working directory to a relative path. A relative path specifies the location of a directory relative to the current working directory. Let’s consider an example:
```python import os relative_directory = "../new_directory" os.chdir(relative_directory) ```
In this example, the os.chdir() function changes the working directory to the directory one level above the current working directory, and then navigates to the new_directory. It’s important to note that the number of ../ in the relative path determines how many levels up you want to go before reaching the desired directory.
By using either absolute or relative paths, you can easily change the working directory in Python, allowing you to organize your files and navigate through different directories effortlessly.
Using absolute and relative paths to change the working directory
In the previous section, we discussed how to change the working directory using both absolute and relative paths. Let’s explore these concepts further and understand when to use each approach.
Absolute paths
Absolute paths provide the complete location of a directory starting from the root directory. These paths are independent of the current working directory and remain consistent regardless of the script’s execution location.
Absolute paths are useful when you want to access files or directories that are located in a fixed location on your system. For example, if you have a configuration file stored in /etc/myapp/config.ini, you can use an absolute path to access it from any working directory.
However, it’s worth noting that absolute paths can be long and cumbersome to type, especially if you have deeply nested directories. In such cases, it might be more convenient to use relative paths.
Relative paths
Relative paths specify the location of a directory relative to the current working directory. These paths are shorter and more concise compared to absolute paths, making them easier to work with.
Relative paths are useful when you want to access files or directories that are located in a specific location relative to your current working directory. For example, if you have a project with the following structure:
my_project/ ├── main.py ├── data/ │ ├── data.csv │ ├── images/ │ │ ├── image1.jpg │ │ └── image2.jpg │ └── results/ └── utils/ ├── helper.py └── config.py
If you are working in the main.py file and want to access the data.csv file, you can use a relative path like data/data.csv to reference it. This way, the code remains portable, and you can easily move the project to a different location without having to update all the file paths.
Relative paths are also useful when you want to navigate through directories without specifying the complete path. For example, if you are in the data directory and want to access the results directory, you can use the relative path ../results. The .. represents the parent directory, allowing you to go up one level before accessing the results directory.
In summary, both absolute and relative paths have their uses, and the choice depends on the specific requirements of your project. Absolute paths provide fixed locations that are independent of the working directory, while relative paths offer more flexibility and portability.
Common errors and troubleshooting when changing working directories
While changing the working directory in Python is relatively straightforward, there are a few common errors that you might encounter. Let’s discuss these errors and how to troubleshoot them.
Directory not found
One common error occurs when the directory you are trying to change to does not exist. If you provide an invalid path or a non-existent directory, Python will raise a FileNotFoundError. To resolve this issue, ensure that the directory exists and the path is correct.
Permission denied
Another common error is the PermissionError, which occurs when you don’t have the necessary permissions to access or change the working directory. To resolve this issue, make sure you have the required permissions or try running the script with elevated privileges.
Invalid characters in the path
Sometimes, the path you provide might contain invalid characters that are not allowed by the operating system. For example, if your path contains special characters or spaces, it can cause errors. To avoid this, ensure that your paths are properly formatted and do not contain any invalid characters.
Using the wrong slashes in the path
On different operating systems, the directory separator can vary. While Windows uses backslashes () as directory separators, Unix-based systems (e.g., Linux, macOS) use forward slashes (/). If you are working on a cross-platform project, it’s essential to use the appropriate directory separator in your paths. To ensure compatibility, you can use the os.path.join() function to join path components using the correct separator.
By being aware of these common errors and troubleshooting steps, you can handle any issues that arise when changing the working directory in Python.
Best practices to manage working directories in Python
Now that you have learned how to get and change the working directory in Python, it’s important to follow some best practices to ensure smooth and efficient file operations. Here are a few tips to help you manage your working directories effectively:
Use context managers
When changing the working directory in Python, it’s good practice to use a context manager to ensure that the working directory is restored to its original state after the required operations are completed. The os module provides the os.chdir() function, but you can also use the pathlib module, which offers a context manager.
```python from pathlib import Path new_directory = Path("/path/to/new/directory") with new_directory: # Perform file operations ```
Using a context manager ensures that the working directory is changed temporarily within the scope of the with block and automatically restored when the block exits.
Use relative paths whenever possible
As mentioned earlier, relative paths offer more flexibility and portability compared to absolute paths. Whenever possible, use relative paths to access files and directories. This allows your code to be more adaptable, as it can be executed from different working directories without needing to update the file paths.
Avoid hardcoding paths
Avoid hardcoding absolute paths directly into your code. Instead, consider using configuration files or environment variables to store paths that may change based on the deployment environment. This allows for easier maintenance and reduces the likelihood of errors when deploying your code to different systems.
Document your file structure
When working on a larger project, it can be helpful to create a document or a diagram that outlines the directory structure and the purpose of each directory. This documentation can serve as a reference for yourself and other developers working on the project, ensuring everyone understands the organization and purpose of each directory.
By following these best practices, you can ensure that your working directories are well-managed, and your code remains maintainable and adaptable to different environments.
Advanced techniques to work with working directory
Now that you have a solid understanding of the basics of getting and changing the working directory in Python, let’s explore some advanced techniques that can further enhance your file operations.
Working with multiple directories
In some cases, you might need to work with multiple directories simultaneously. Python provides the os.path module, which offers various functions for manipulating file paths. One such function is os.path.join(), which allows you to join multiple path components into a single path.
```python import os directory_1 = "/path/to/directory1" directory_2 = "directory2" file_name = "myfile.txt" path = os.path.join(directory_1, directory_2, file_name) ```
In the above example, the os.path.join() function is used to create a path that combines directory_1, directory_2, and file_name. This approach is more robust than manually concatenating strings, as it takes care of handling the appropriate directory separators.
Using pathlib for path manipulation
In addition to the os.path module, Python provides the pathlib module, which offers an object-oriented approach to path manipulation. The pathlib.Path class allows you to perform various operations on file paths, such as joining paths, checking file existence, and retrieving file attributes.
```python from pathlib import Path path = Path("/path/to/directory/myfile.txt") parent_directory = path.parent file_name = path.name ```
In the above example, the Path class is used to create a path object for the file myfile.txt. You can then access various attributes of the path, such as the parent directory and the file name.
The pathlib module provides a more intuitive and readable API compared to the os.path module. It’s a powerful tool for working with file paths and highly recommended for advanced path manipulation.
Third-party libraries to manage working directories
While Python provides built-in modules like os and pathlib for managing working directories, there are also third-party libraries available that offer additional functionality and convenience. Let’s explore two popular libraries: click and pathlib2.
Click
Click is a popular third-party library for creating command-line interfaces in Python. It provides a powerful and intuitive way to handle command-line arguments, including file and directory paths.
```python import click @click.command() @click.argument("directory", type=click.Path(exists=True)) def my_command(directory): click.echo(f"You selected directory: {directory}") if name == "main": my_command() ```
In the above example, the click.Path argument type is used to specify that the argument should be a valid path. Additionally, the exists=True parameter ensures that the specified path exists.
Using click makes it easy to handle file and directory paths as command-line arguments, providing a seamless integration with your Python scripts.
Pathlib2
Pathlib2 is a backport of the pathlib module for Python 2. It provides the same functionality as pathlib but can be used in older Python versions.
```python from pathlib2 import Path path = Path("/path/to/directory/myfile.txt") parent_directory = path.parent file_name = path.name ```
With pathlib2, you can use the same object-oriented approach to path manipulation in Python 2, ensuring compatibility with older codebases.
Conclusion and final thoughts
In this article, we have explored the importance of understanding and changing the working directory in Python. We have learned how to get the current working directory and how to change it using both absolute and relative paths. We have also discussed best practices, common errors, and advanced techniques for working with working directories.
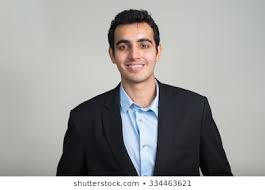
Nishant Verma is a senior web developer who love to share his knowledge about Linux, SysAdmin, and more other web handlers. Currently, he loves to write as content contributor for ServoNode.