Are you looking to reverse a string in Python? Well, you’ve come to the right place! Reversing a string is a common task in programming, and Python provides an elegant way to accomplish this.
Basic string manipulation in Python
Before we dive into the different methods for reversing a string in Python, let’s first explore some basic string manipulation techniques. In Python, a string is an immutable sequence of characters, which means that once a string is created, it cannot be changed. However, we can perform various operations on strings to manipulate them.
One of the most common string manipulation operations is concatenation, which allows us to combine two or more strings into a single string. For example, we can concatenate the strings “Hello” and “World” to create the string “Hello World”.
Another useful operation is string slicing, which allows us to extract a portion of a string. This is done by specifying the start and end indices of the desired substring. For example, if we have the string “Hello World”, we can use slicing to extract the substring “World” by specifying the indices [6:11].
In addition to concatenation and slicing, Python provides a wide range of built-in string methods that allow us to perform various operations on strings, such as converting them to uppercase or lowercase, replacing substrings, and splitting strings into lists of words.
Understanding string slicing and indexing in Python
Now that we have a basic understanding of string manipulation in Python, let’s explore how we can use string slicing and indexing to reverse a string. String slicing allows us to extract a portion of a string by specifying the start and end indices. By using a negative step value in the slice, we can reverse the order of characters in a string.
To reverse a string using string slicing, we can specify a step value of -1 in the slice. This will start from the last character of the string and move towards the first character, effectively reversing the order of characters. For example, if we have the string “Hello”, we can use slicing with a step value of -1 to obtain the reversed string “olleH”.
In addition to string slicing, we can also use string indexing to access individual characters in a string. In Python, strings are zero-indexed, which means that the first character has an index of 0, the second character has an index of 1, and so on. By using negative indices, we can access characters from the end of the string. For example, the last character of a string can be accessed using the index -1, the second-to-last character using the index -2, and so on.
Implementing the reverse function in Python
Now that we understand the basics of string slicing and indexing, let’s implement a function that can reverse a given string in Python. This function will take a string as input and return the reversed string as output.
python def reverse_string(input_string): return input_string[::-1]
In the above code, we define a function called reverse_string that takes a single parameter input_string. We use string slicing with a step value of -1 to reverse the order of characters in the input string and return the result.
Testing the reverse function with sample strings
To ensure that our reverse_string function works correctly, let’s test it with some sample strings. We will pass different strings as input to the function and verify that the output is the reverse of the input.
python print(reverse_string("Hello")) # Output: "olleH" print(reverse_string("Python")) # Output: "nohtyP" print(reverse_string("12345")) # Output: "54321"
By executing the above code, we can confirm that our reverse_string function is indeed reversing the input strings correctly.
Handling special cases and edge cases
While our reverse_string function works well for most cases, there are some special cases and edge cases that we need to consider. For example, what should happen if the input string is empty? In this case, we can simply return an empty string as the output.
Another edge case to consider is when the input string contains only one character. In this case, the reversed string will be the same as the input string. We can handle this case by checking if the length of the input string is equal to 1, and if so, returning the input string as is.
Additionally, we should also handle cases where the input string contains whitespace characters. In these cases, we need to ensure that the whitespace characters are preserved in the reversed string. One way to achieve this is by using the join() method to concatenate the reversed characters with an empty string, preserving any whitespace characters.
Time & space complexity analysis of string reversal
Now that we have implemented a function to reverse a string in Python, let’s analyze its time and space complexity. The time complexity of our reverse_string function is O(n), where n is the length of the input string. This is because we need to iterate over each character in the input string to reverse it.
As for the space complexity, our reverse_string function has a space complexity of O(n) as well. This is because we create a new string that is the same length as the input string to store the reversed characters.
Alternative approaches to string reversal in Python
While string slicing is a simple and efficient way to reverse a string in Python, there are alternative approaches that can be used as well. One such approach is to use the built-in reversed() function, which returns an iterator that produces the characters of a string in reverse order.
To reverse a string using the reversed() function, we can convert the iterator to a string by using the join() method, specifying an empty string as the separator. This will concatenate the characters in reverse order, effectively reversing the string.
Here’s an example of how to use the reversed() function to reverse a string:
python input_string = "Hello" reversed_string = ''.join(reversed(input_string)) print(reversed_string) # Output: "olleH"
By executing the above code, we can see that the reversed() function produces the same result as our reverse_string function.
Best practices for using the reverse function in Python
When using the reverse function in Python, there are a few best practices that we should keep in mind. Firstly, it is important to consider the performance implications of reversing a string, especially if the string is large. Reversing a string requires iterating over each character, so the time complexity is linear with respect to the length of the string. If performance is a concern, it may be worth exploring alternative approaches or optimizations.
Another best practice is to handle special cases and edge cases appropriately. As mentioned earlier, we should consider cases where the input string is empty, contains only one character, or contains whitespace characters. By handling these cases explicitly, we can ensure that our reverse function behaves as expected in all scenarios.
Finally, it is recommended to write clear and concise code with proper documentation and comments. This will make our code more readable and maintainable, and will also help other developers understand our intentions and any potential limitations.
Conclusion and final thoughts
Reversing a string in Python is a common task that can be accomplished using various techniques. In this article, we explored the basics of string manipulation in Python, including string slicing and indexing. We also implemented a function to reverse a string using string slicing, and discussed alternative approaches using the reversed() function.
When using the reverse function in Python, it is important to consider performance implications and handle special cases and edge cases appropriately. By following best practices and writing clear and concise code, we can ensure that our reverse function is efficient, robust, and easy to understand.
So whether you’re a beginner or an experienced Python developer, these techniques for reversing a string in Python will undoubtedly come in handy for your projects. Happy coding!
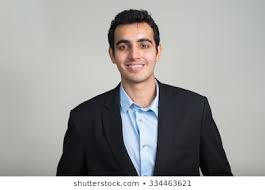
Nishant Verma is a senior web developer who love to share his knowledge about Linux, SysAdmin, and more other web handlers. Currently, he loves to write as content contributor for ServoNode.