Welcome to the world of Linux scripting! If you’re a Linux enthusiast or a beginner learning shell scripting, understanding the if..else statement is essential. This powerful construct allows you to control the flow of your scripts based on conditions you define.
In this article, we’ll dive into the bash if..else statement in Linux and explore its syntax and usage through practical examples. Whether you’re a sysadmin automating tasks or a developer creating complex scripts, mastering if..else statements will greatly enhance your scripting skills.
Syntax of the Bash if..else statement
The syntax of the bash if..else statement in Linux is straightforward and easy to understand. It follows the basic structure:
bash if [ condition ] then # Code to be executed if the condition is true else # Code to be executed if the condition is false fi
The if keyword is followed by a condition enclosed in square brackets ([ and ]). If the condition evaluates to true, the code within the then block is executed. If the condition evaluates to false, the code within the else block is executed. The fi keyword marks the end of the if..else statement.
Basic if..else statement example
Let’s start with a basic example to demonstrate the usage of the if..else statement. Suppose we want to check if a number is positive or negative. We can write a bash script like this:
```bash !/bin/bash read -p "Enter a number: " num if [ $num -gt 0 ] then echo "The number is positive" else echo "The number is negative" fi ```
In this example, we use the read command to prompt the user to enter a number. The value is stored in the num variable. The if..else statement checks if the value of num is greater than 0. If it is, the script prints “The number is positive.” Otherwise, it prints “The number is negative.”
Nested if..else statement example
The bash if..else statement can also be nested to handle more complex conditions. Let’s consider a scenario where we want to determine if a number is positive, negative, or zero. We can achieve this by using a nested if..else statement:
```bash !/bin/bash read -p "Enter a number: " num if [ $num -gt 0 ] then echo "The number is positive" elif [ $num -lt 0 ] then echo "The number is negative" else echo "The number is zero" fi ```
In this example, we add an elif statement to handle the case when the number is zero. If the number is greater than 0, the script prints “The number is positive.” If the number is less than 0, it prints “The number is negative.” And if the number is equal to 0, it prints “The number is zero.”
Using logical operators in if..else statements
The bash if..else statement can also handle more complex conditions using logical operators such as && (AND), || (OR), and ! (NOT). These operators allow you to combine multiple conditions to create more sophisticated logic in your scripts.
Here’s an example that demonstrates the usage of logical operators in if..else statements:
```bash !/bin/bash read -p "Enter your age: " age if [ $age -ge 18 ] && [ $age -le 65 ] then echo "You are eligible to work" else echo "You are not eligible to work" fi ```
In this example, we use the logical && operator to combine two conditions. The first condition checks if the age is greater than or equal to 18, and the second condition checks if the age is less than or equal to 65. If both conditions evaluate to true, the script prints “You are eligible to work.” Otherwise, it prints “You are not eligible to work.”
Example of using logical operators in if..else statements
Let’s consider another example that showcases the usage of logical operators in if..else statements. Suppose we want to check if a number is divisible by both 2 and 3. We can write a bash script like this:
```bash !/bin/bash read -p "Enter a number: " num if [ $((num % 2)) -eq 0 ] && [ $((num % 3)) -eq 0 ] then echo "The number is divisible by both 2 and 3" else echo "The number is not divisible by both 2 and 3" fi ```
In this example, we use the modulo operator % to check if the remainder of dividing the number by 2 and 3 is equal to 0. If both conditions evaluate to true, the script prints “The number is divisible by both 2 and 3.” Otherwise, it prints “The number is not divisible by both 2 and 3.”
Case statement: alternative to if..else statement
While the bash if..else statement is powerful and versatile, there are cases where the case statement can be a more suitable alternative. The case statement allows you to perform different actions based on the value of a variable or expression.
Here’s an example that demonstrates the usage of the case statement in Bash:
```bash !/bin/bash read -p "Enter a color: " color case $color in red) echo "You chose red" ;; green) echo "You chose green" ;; blue) echo "You chose blue" ;; *) echo "Invalid color" ;; esac ```
In this example, the case statement checks the value of the color variable. If the value is “red,” it prints “You chose red.” If the value is “green,” it prints “You chose green.” If the value is “blue,” it prints “You chose blue.” If the value does not match any of the specified patterns, it prints “Invalid color.”
Common errors and troubleshooting tips
When working with if..else statements in bash, there are a few common errors that you may encounter. Here are some troubleshooting tips to help you overcome these issues:
- Syntax errors: Make sure you have the correct syntax for the if..else statement, including the proper placement of brackets, keywords, and semicolons.
- Variable expansion: When using variables in conditions, make sure to properly expand them with the $ symbol.
- String comparisons: If you’re comparing strings, use the = operator instead of the -eq operator used for numerical comparisons.
- Indentation: While indentation is not required in bash scripts, it can greatly improve code readability and help you spot errors more easily.
By being aware of these common pitfalls and following best practices, you can avoid many issues when working with if..else statements in bash.
Conclusion
The bash if..else statement is a fundamental construct in Linux scripting that allows you to control the flow of your scripts based on conditions you define. By mastering if..else statements, you can write powerful and efficient bash scripts that automate tasks and handle complex logic.
In this article, we explored the syntax of the bash if..else statement and showcased various examples to demonstrate its usage in real-world scenarios. We also discussed the usage of logical operators and the case statement as alternatives to if..else statements. Additionally, we provided troubleshooting tips to help you overcome common errors.
Now that you have a solid understanding of if..else statements in Linux, it’s time to apply your knowledge and take your scripting skills to the next level. Start experimenting with different conditions and scenarios, and see how if..else statements can enhance your Linux scripting capabilities. Happy scripting!
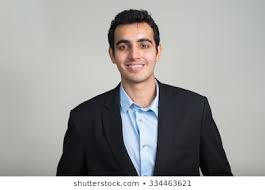
Nishant Verma is a senior web developer who love to share his knowledge about Linux, SysAdmin, and more other web handlers. Currently, he loves to write as content contributor for ServoNode.