access command in Linux is basically used to check if the calling program has access to an specific file, or it checks if the file is in existence or not. This can be accomplished by utilisation of calling process’s UID and GID. In case if the specifiedpathname is symbolic link, it’s dereferenced. Below is how to use access command with syntax:
int access(const char *pathname, int mode);
in the above syntax, const char *pathname refers to specified file’s path while the int mode is referred as flags which are mostly used as:
- F_OK : Used for checking existence of file
- R_OK : Checks the file for read permissions
- W_OK : Checks the file for write permissions
- X_OK : Checks the file for execute permissions
However, in case if the access command fails to access the file, it will return the value as -1, otherwise 0 indicating to success.
Example: In the example below, we have used F_OK flag to check if the file is existing or not. Obviously, if the file is found, it will return 0 else -1 as result.
clude<stdio.h> #include<unistd.h> #include<errno.h> #include<sys/types.h> #include<sys/stat.h> #include<fcntl.h> extern int errno; int main(int argc, const char *argv[]){ int fd = access("sample.txt", F_OK); if(fd == -1){ printf("Error Number : %d\n", errno); perror("Error Description:"); } else printf("No error\n"); return 0; }
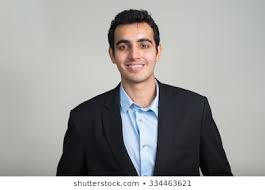
Nishant Verma is a senior web developer who love to share his knowledge about Linux, SysAdmin, and more other web handlers. Currently, he loves to write as content contributor for ServoNode.